Search
Python Tuples
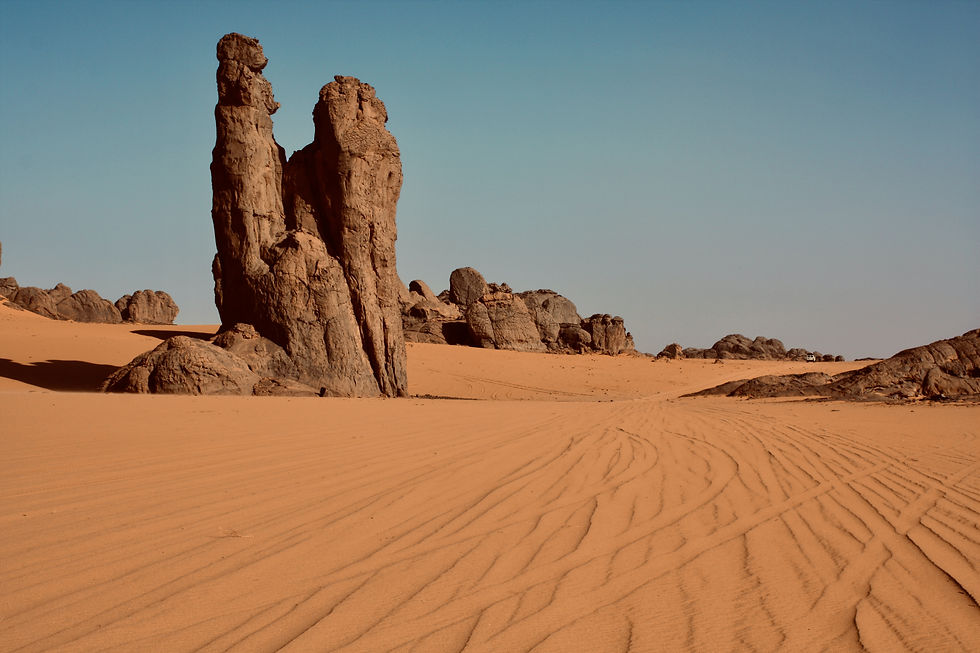
Tuples store multiple items in a single variable. Tuples are immutable: values inside a tuple cannot be updated or changed.
Initializing tuples
Place elements inside parenthesis separated by comma.
Syntax: tuple = ( element1, element2, .... )
##Initialize some tuples
tuple_empty = ()
tuple_num = (1, 8, 9)
--------------------------------------------------------------------------
##Print the content of the tuples
print(tuple_empty)
: ()
print(tuple_num)
: (1, 8, 9)
--------------------------------------------------------------------------
##Print the data type
print(type(tuple_empty))
: <class 'tuple'>
print(type(tuple_num))
: <class 'tuple'>
Accessing items of a tuple
Tuples are ordered data structure which means we can use indexing to retrieve a specific element.
If you are not familiar with the concept of indexing, check out my post on lists HERE.
##Initialize a tuple
nums = (1, 3, 5, 8, 10, 15, 16)
##To access the first, third and last element:
print(nums[0])
: 1
print(nums[2])
: 5
print(nums[-1])
: 16
##To access the first 5 elements:
first_five = nums[:5]
print(first_five)
: (1, 3, 5, 8, 10)
##To access the last 3 elements:
last_two = nums[-2:]
print(last_two)
: (15, 16)
##To access integers from 3 to 15:
middle = nums[1:-1]
print(middle )
: (3, 5, 8, 10, 15)
Tuples are immutable
If we attempt to change the values of a tuple an error is thrown.
##Initialize a tuple
nums = (1, 3, 5, 8)
##Change 3 to 10
nums[1] = 10
: TypeError: 'tuple' object does not support item assignment
Common methods with tuples
##Initialize a tuple
nums = (1, 3, 5, 8)
--------------------------------------------------------------------------
##Length of tuple --> len()
length_t = len(nums )
print(length_t)
: 4
--------------------------------------------------------------------------
##Concatenate 2 tuples together:
nums1 = (1, 3)
nums2 = (0, 5, 8)
all_num = nums1 + nums2
print(all_num)
: (1, 3, 0, 5, 8)
--------------------------------------------------------------------------
##Nesting tuples:
nums1 = ('a', 'b', 'c')
nums2 = (0, 5, 8)
nested_num = (nums1, nums2)
print(nested_num)
: (('a', 'b', 'c'), (0, 5, 8))
#To access the first item of the second tuple:
print(nested_num[1][0])
: 0
--------------------------------------------------------------------------
##Check if an element is present in a tuple:
lett = ('a', 'b', 'c', 'd')
#Does lett contain 'a'
print('a' in lett)
: True
#Does lett contain 'f'
print('f' in lett)
: False
---------------------------
##Return the number of times a value appears in a tuple --> count()
nums = (5, 0, 8, 8, 10)
print(nums.count(8))
: 2
--------------------------------------------------------------------------
##Return the position of the first occurrence of the value --> index()
nums = (5, 0, 8, 8, 10)
print(nums.index(0))
: 1
print(nums.index(10))
: 4
--------------------------------------------------------------------------
##Other functions max(), min(), sum(), sorted()
nums = (5, 0, 8)
print(max(nums)) #max value
: 8
print(min(nums)) #min value
: 0
print(sum(nums)) #sum of all values
: 13
print(sorted(nums)) #sort the elements of the tuple
: (0, 5, 8)
Concluding remarks
Because tuples are immutable, they tend to used less compared to lists. Tuples share with lists several methods, but tuples are faster than lists and make the code much safer. Tuples are normally implemented when we need to store information that must never be changed.
Comments