Search
Python Sets
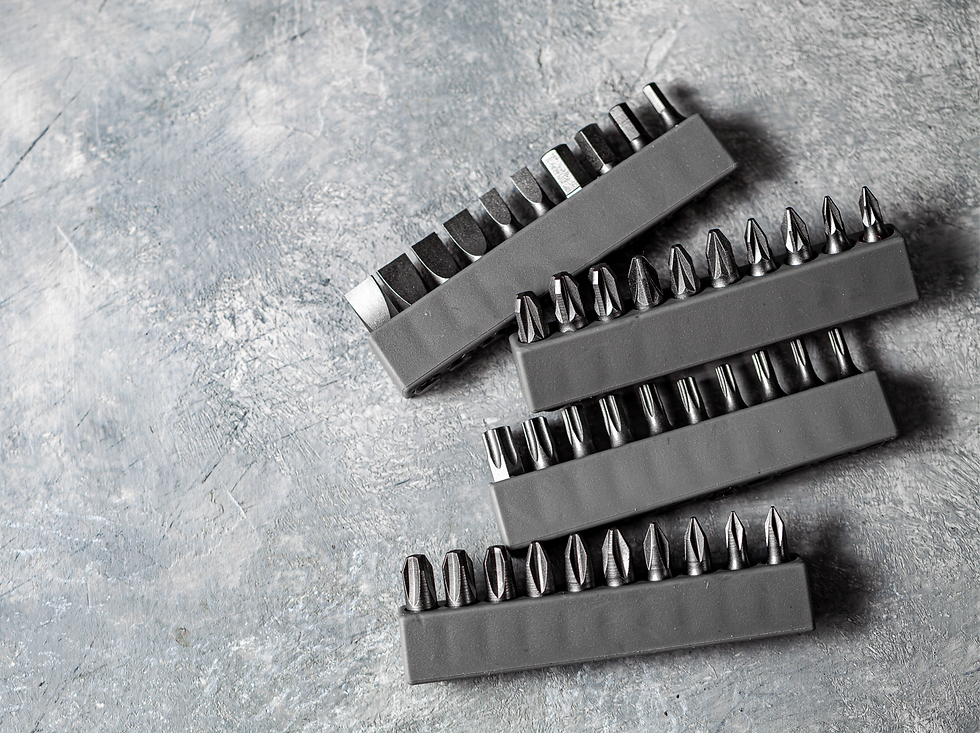
Sets are used to store multiple UNIQUE items in a single variable. Sets are unordered data structure.
Initializing sets
Place items inside curly brackets separated by comma.
Syntax: set = { element1, element2, .... }
##Initialize some sets
set1 = {} #Empty set
set2 = {1, 2, 3}
set2 = {4, 8, 'r'}
--------------------------------------------------------------------------
##Print the content of the set
print(set1)
: {}
print(set2)
: {1, 2, 3}
print(set3)
: {4, 8, 'r'}
--------------------------------------------------------------------------
##Check the type:
print(type(set1))
: <class 'set'>
--------------------------------------------------------------------------
##Sets are collections of unique elements
set_num = {1, 2, 3, 2, 1}
print(set_num)
: {1, 2, 3} #--> only unique elements are stored
Common methods & functions with sets
##To add the element to the set --> add()
set_num = {1, 2, 3}
set_num.add(20) #Add the integer 20
print(set_num)
: {1, 2, 3, 20}
--------------------------------------------------------------------------
##To remove a value from set --> remove() #Option 1
set_num.remove(2) #Remove the integer 2
print(set_num)
: {1, 3, 20}
##To remove a value from set --> discard() #Option 2
set_num.discard(3) #Remove the integer 3
print(set_num)
: {1, 20}
##What's the difference between remove() and discard()?
#--> remove() WILL throw an error if the item we are trying to remove is #non present in the set
#--> discard() WON'T throw an error if the item we are trying to remove #is non present in the set
set_num = {1, 20, 8}
set_num.discard(5) #No error is thrown
set_num.remove(5) #TypeError is thrown
: TypeError: 5
--------------------------------------------------------------------------
##To add multiple values to a set --> update()
set_num = {1, 20, 8, 10}
set_num.update([22, 85]) #Add 22 and 85 to the set
print(set_num)
: {1, 8, 10, 20, 85, 22}
Main operations with sets
These are Union, Intersection, Difference:
##Initialize two sets
set1 = {1, 20, 8, 10}
set2 = {88, 8, 10, 12}
--------------------------------------------------------------------------
##UNION: merge the elements of two sets into one set --> union() or |
set_final = set1.union(set2) #Syntax option 1
print(set_final)
: {1, 8, 10, 12, 20, 88}
set_final2 = set1 | set2 #Syntax option 2
print(set_final2)
: {1, 8, 10, 12, 20, 88}
--------------------------------------------------------------------------
##INTERSECTION: Find common elements from two sets --> intersection() or &
set_intersect1 = set1.intersection(set2) #Syntax option 1
print(set_intersect1)
: {8, 10}
set_intersect2 = set1 & set2 #Syntax option 2
print(set_intersect2)
: {8, 10}
--------------------------------------------------------------------------
##DIFFERENCE: Find difference of elements from one set to the second set
## --> difference() or -
set_difference1 = set1.difference(set2) #Syntax option 1
print(set_difference1)
: {1, 20}
set_difference2 = set1 - set2 #Syntax option 1
print(set_difference2)
: {1, 20}
# NOTE set1 - set2 != set2 - set1
set1_minus_set2 = set1 - set2
set2_minus_set1 = set2 - set1
print(set1_minus_set2 , set2_minus_set1)
print(set1_minus_set2 == set2_minus_set1)
: {1, 20} {12, 88}
: False
Identity between sets
Two sets are identical if they contains the same UNIQUE items
set1 = {1, 8, 10}
set2 = {8, 8, 10, 1}
print(set1 == set2)
: True
Lists to sets and backward
Lists can be converted to sets and vice versa. Lists are often converted to sets to remove duplicated items.
##Initialize a list with duplicated elements
nums = [8, 8, 10, 1]
print(type(nums))
: <class 'list'>
#Convert the list to a set to remove duplicates
nums_set = set(nums)
#Convert the set back to a list
unique_num_list = list(nums_set)
print(unique_num_list)
: [8, 1, 10]
print(type(unique_num_list))
: <class 'list'>
Use case
Given a list of unique and duplicated integers, create a list containing only its unique squares.
##Initialize a list with duplicated elements
nums = [1, 3, 7, 7, 3, 8, 9, 10]
#Create a list containing the squares
square_nums = []
for num in nums:
square_nums.append(num**2)
#Remove the duplicated squares
result = list(set(square_nums))
print(result)
: [64, 1, 100, 9, 81, 49]
Concluding remarks
Sets are optimized for checking whether a specific element is present or not in the set. Sets are unordered data structure, thus we can't access their item by their position/index.
Comentários