Python Basic Data Types
- Gianluca Turcatel
- Dec 21, 2021
- 7 min read
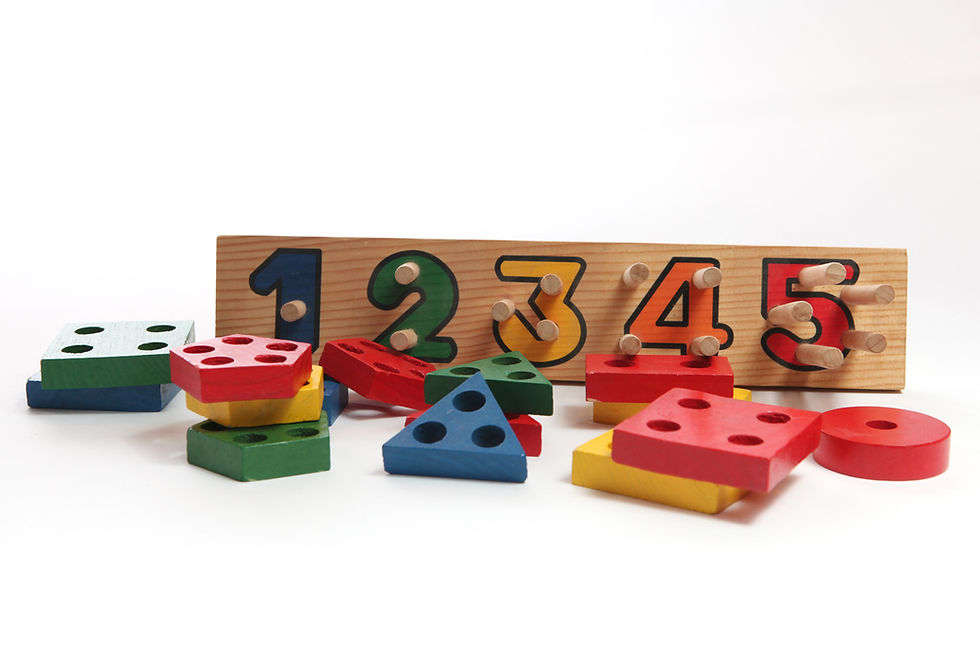
Python basic data types include the integer, float, string and boolean.
Integers
Integers are positive and negative integer numbers that are written without a fractional component. Basically, every number without a decimal point.
##Example of integers
num1 = 2
num2 = 5
#Print num1, num2
print(num1)
: 2
print(num2)
: 5
#Check the data type of num1, num2
print(type(num1))
: <class 'int'>
print(type(num2))
: <class 'int'>
Floats
Integers are positive and negative integer numbers with a decimal point dividing the integer and the fractional parts.
##Example of floats
num1 = 2.0
num2 = 5.8
num3 = 3. #Adding . tells Python that's a float
#Print num1, num2, num3
print(num1)
: 2.0
print(num2)
: 5.8
print(num3)
: 3.0
#Check the data type of num1, num2, num3
print(type(num1))
<class 'float'>
print(type(num2))
: <class 'float'>
print(type(num3))
: <class 'float'>
Operations with integers and floats
##Multiplication --> *
print(6 * 5)
:30
print(2.1 * 5)
: 10.5
--------------------------------------------------------------------------
##Sum --> +
print(6 + 5)
:11
print(2.1 + 5)
: 7.1
--------------------------------------------------------------------------
##Division --> /
print(10 / 5) #Note: division between two integers generates a float
: 2.0
print(type(10 / 5)) #Confirm the data type
: <class 'float'>
print(5.5 / 5)
: 1.1
--------------------------------------------------------------------------
##Difference --> -
print(10 - 5)
: 5
print(5.5 - 5)
: 0.5
--------------------------------------------------------------------------
##Exponentiation --> **
print(10 ** 2)
: 100
print(1.5 ** 2)
: 2.25
--------------------------------------------------------------------------
##Module aka remainder of a division --> %
print(10 % 2)
: 0
print(5.5 % 2)
: 0.5
Converting integers to floats and vice versa
##Convert float to integer --> int()
print(int(2.3))
: 2
print(int(2.8)) #Note: int() does not round numbers as you would expect
: 2
print(type(int(2.8)))
: <class 'int'>
--------------------------------------------------------------------------
##Convert integer to float --> float()
print(float(2))
: 2.0
print(type(float(2)))
: <class 'float'>
Strings
Strings are every alphanumerical character or series of characters surrounded by single quotation marks or double quotation marks.
##Example of strings
name = 'Mike'
city = "San Diego"
component = "ID00025"
empty = "" #Empty string
#Print the 4 strings:
print(name)
: Mike
print(city)
: San Diego
print(component)
: ID00025
print(empty)
:
#Check data type:
print(type(name))
: <class 'str'>
print(type(city))
: <class 'str'>
print(type(component))
: <class 'str'>
print(type(empty))
: <class 'str'>
String slicing
To extract a single characters or part of a string we leverage the concept of indexing: each character of a string is identified by its location (index). Indexing in Python starts from zero, which means the first character has index 0, the second character has index 1 and so on. The highest index of a string is equal to length of the string minus 1.
- Syntax for extracting a single character: char = string[index]
- Syntax for extracting part (slice) of as string: char = string[start_index : end_index ]
Note: the item with the start index WILL BE sliced by Python; the item with the end index
WON'T BE sliced by Python. Therefore to slice a string until the character with index n included,
we must pass n +1 as end_index
#Index representation
#Index 012345678
city = "San Diego"
--------------------------------------------------------------------------
##Access single characters
char1 = city[4] #Letter D which is the fifth character, thus index 4
print(char)
: D
char2 = city[0] #Letter S which is the first character, thus index 0
print(char2)
: S
char3 = city[3] #White space which is the forth character, thus index 3
print(char3)
:
char_last = city[8] #The last character. Option 1: use index 8 (length-1)
print(char_last)
: o
char_last = city[-1] #The last character. Option 2: use index -1
print(char_last)
: o
char_last = city[-2] #The character before the last one: index -2
print(char_last)
: g
--------------------------------------------------------------------------
#Index 012345678
city = "San Diego"
##Access part of a string: slicing
chars = city[1:5] #Letters a (index 1) to D (index 4) included
print(chars)
: an D
chars1 = city[0:5] #First 5 characters 'San D' - option 1
print(chars1)
: an D
chars1 = city[:5] #First 5 characters 'San D' - option 2
print(chars1)
: an D
chars2 = city[7:9] #Last 2 characters 'go' - option 1
print(chars2)
: go
chars2 = city[-2:] #Last 2 characters 'go' - option 2
print(chars2)
: go
Common methods with strings - basics
##Initialize few strings:
name = 'Mike'
city = "San Diego"
empty = ""
--------------------------------------------------------------------------
##Length of a string --> len()
print(len(name))
: 4
print(len(city)) #Note: the white space contributes to the string's length
: 9
print(len(empty))
: 0
--------------------------------------------------------------------------
##Check if a(some) character(s) is(are) present in a string --> in
print('a' in city)
: True
print('a' in name)
: False
print('San' in city)
: True
--------------------------------------------------------------------------
##Concatenating strings together --> +
name = 'Mike'
last_name = 'Smith'
full_name = name + '_' + last_name
print(full_name)
: Mike_Smith
--------------------------------------------------------------------------
##Concatenating a string n times --> *
greeting = 'hello'
multiple_greeting = greeting * 4
print(multiple_greeting)
: hellohellohellohello
Common methods with strings - advanced
##Initialize a string:
full_name_age_sex = 'Mike Smith, 30, Male'
--------------------------------------------------------------------------
##To convert all characters to lowercase --> lower()
full_name_age_sex_lower = full_name_age_sex.lower()
print(full_name_age_sex_lower)
: mike smith, 30, male
--------------------------------------------------------------------------
##To convert all characters to uppercase --> upper()
full_name_age_sex_upper = full_name_age_sex.upper()
print(full_name_age_sex_upper)
: 'MIKE SMITH, 30, MALE'
--------------------------------------------------------------------------
##To split the string on a specific character --> split()
split_w = full_name_age_sex.split() # by default it splits on white spaces
print(split_w)
: ['Mike', 'Smith,', '30,', 'Male'] # The output is list of strings
#To split on the character 'm' (lowercase 'm')
splitted_m = full_name_age_sex.split('m') # 'm' is passed as argument
print(splitted_m)
: ['Mike S', 'ith, 30, Male']
--------------------------------------------------------------------------
##To count the times a character (or sequence of characters) appears in a #string --> count()
long_string = 'Your payment was been posted tonight.'
print(long_string.count('o')) #Count 'o' in the string
: 3
print(long_string.count('pay')) #Count 'pay' in the string
: 1
#Count 'o' in the first 20 (0, 20) characters of the string
print(long_string.count('o', 0, 20))
: 1
Conversion between strings and integers/float
Converting integers and float to strings is always successful and a valid thing to do. However, strings can be converted to integer and floats if they ONLY contain numerical characters (and . for floats).
##Integer to string --> str()
print(str(1))
: 1
print(type(str(1)))
: <class 'str'>
--------------------------------------------------------------------------
##String to integer --> int()
print(int('56')) #Valid conversion
: 56
print(type(int('56'))) #Valid conversion
: <class 'int'>
print(int('56h')) #Not valid conversion
: ValueError: invalid literal for int() with base 10: '56h'
--------------------------------------------------------------------------
##Float to string --> str()
print(str(3.5))
: 3.5
print(type(str(3.5)))
: <class 'str'>
--------------------------------------------------------------------------
##String to float --> float()
print(float('23.5')) #Valid conversion
: 23.5
print(type(float('23.5'))) #Valid conversion
: <class 'float'>
print(float('F23.5')) #Not valid conversion
: ValueError: could not convert string to float: 'F23.5'
Booleans
Booleans represent the truth value of an expression. A Boolean variable can be either True or False.
##The two booleans:
bool1 = True
bool2 = False
#Print bool1 , bool2:
print(bool1 , bool2)
: True False
#Check the data type of bool1 , bool2:
print(type(bool1))
: <class 'bool'>
print(type(bool2))
: <class 'bool'>
Use of booleans
##To compare variables or check if a condition is met:
age = 30
age_above_18 = age > 18
print(age_above_18)
: True
name = 'Mike'
print(name == 'Mike')
: True
Conversion between booleans and integers/floats
Interestingly, we can convert booleans to integers (floats). The conversion rule is:
True = 1 (1.0)
False = 0 (0.0)
bool1 = True
bool2 = False
--------------------------------------------------------------------------
##To convert booleans to integers --> int()
bool1_int = int(bool1)
bool2_int = int(bool2)
print(bool1_int, bool2_int)
: 1 0
--------------------------------------------------------------------------
##To convert booleans to floats --> float()
bool1_float = float(bool1)
bool2_float = float(bool2)
print(bool1_float, bool2_float)
: 1.0 0.0
We can convert integers and floats to boolens. The conversion rule is:
0, 0.0 = False
All other integers and floats = True
##To convert floats and integers to booleans --> bool()
print(bool(0))
: False
print(bool(0.))
: False
print(bool(5))
: True
print(bool(-5.2))
: True
print(bool(1))
: True
Conversion between booleans and strings
Booleans can be easily converted to strings. Strings can be converted to booleans as well, but the result of the conversion might not be as what you would expect.
##To convert booleans to strings --> str()
bool1 = True
bool2 = False
bool1_str = str(bool1)
bool2_str = str(bool2)
print(bool1_str, bool2_str)
: True False
print(type(bool1_str), type(bool2_str))
: <class 'str'> <class 'str'>
--------------------------------------------------------------------------
##To convert strings to booleans --> bool()
string1 = 'True'
print(bool(string1))
: True #We expected that!
string2 = 'False'
print(bool(string2))
: True #Surprise?
string3 = 'hello'
print(bool(string3))
: True #More surprise?
string4 = '' #Empty string
print(bool(string4))
: False
#RULE: empty string is converted to False. Every other string to True
The conversion from string to boolean does not make sense most of the times. For instance, we would want that 'FALSE', 'False', 'false', 'no' and so on, to be converted to the boolean False. Python provides a function called strtobool that computes these semantic conversions and returns 0 for False and 1 for True.
from distutils.util import strtobool #Import the function
print(strtobool('True'))
print(strtobool('TRUE'))
print(strtobool('YES'))
print(strtobool('yes'))
print(strtobool('on'))
: 1
: 1
: 1
: 1
: 1
print(strtobool('False'))
print(strtobool('FALSE'))
print(strtobool('No'))
print(strtobool('off'))
print(strtobool('n'))
: 0
: 0
: 0
: 0
: 0
Conversion between booleans and other Python data types
This will be fun.
print(bool([])) #Empty list to boolean
: False
print(bool([1])) #Non empty list to boolean
: True
print(bool(set())) #Empty set to boolean
: False
print(bool({1})) #Non empty set to boolean
: True
print(bool(())) #Empty tuple to boolean
: False
print(bool((1))) #Non empty tuple to boolean
: True
You probably see the trend: empty sequences and collections are False; True otherwise.
Operations with booleans
##Operation AND
True and True = True #Alternative syntax: True & True
True and False= False #Alternative syntax: True & False
False and True = False #Alternative syntax: False & True
False and False = False #Alternative syntax: False & False
--------------------------------------------------------------------------
##Operation OR
True or True = True #Alternative syntax: True | True
True or False= True #Alternative syntax: True | False
False or True = True #Alternative syntax: False | True
False or False = False #Alternative syntax: False | False
--------------------------------------------------------------------------
##Examples with AND, OR
age = 30
income = 85000
print(age > 18 & income > 80000 )
: True
print((age > 18 & income > 100000) | (age < 18 & income < 80000))
: False
--------------------------------------------------------------------------
##Sum, Multiplication, difference, division: we can sum/multiply/subtract #/divide booleans together (False = 0, True = 1)
print(True + True + True)
: 3
print((True + True) * True)
: 2
print((True + True + False) * False)
: 0
print((True / True + False) - True )
: 0
Concluding remarks
In this article we covered the basics and some advanced properties of the four Python basic data types. Each data type has specific methods and features. Conversion between Python data types is generally allowed but sometimes the result of the conversion is unexpected.
Comments