Python Lists
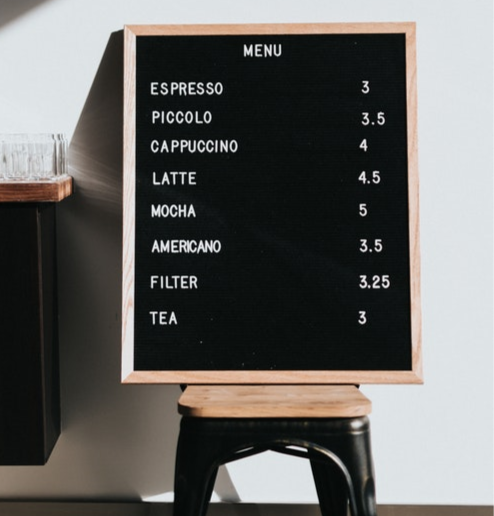
Lists are probably the most widely used data structure in Python. Lists store multiple items in a single variable. Moreover, lists are ordered data structure: the order on which the elements of a list are arranged is as important as the elements themselves.
Initializing lists
Place items inside squared brackets separated by comma.
Syntax: list = [ element1, element2, .... ]
##Initialize some lists
list1 = [] #empty list
nums = [1, 2, 3, 4] #list of integers
letters = ['a', 'b', 'c'] #list of characters
--------------------------------------------------------------------------
##Print the content of the lists
print(list1)
: []
print(nums)
: [1, 2, 3, 4]
print(letters)
: ['a', 'b', 'c']
Lists are ordered data structures
If two lists contains the same elements but the order is different, the two lists are different.
##Initialize 2 lists
list1 = [1,2,3,4]
list2 = [2,1,3,4]
--------------------------------------------------------------------------
##Compare the 2 lists
print(list1 == list2 )
: False
Accessing items of a list - basics
We leverage the concept of indexing: referring to an element of a list by its position within the list. Items inside a list are indexed starting from 0: the first element of the list has index 0, the second index 1 and so on. Note that the highest index for a list is equal to the number of items withing a list minus 1.
To access an element of list, the element's index is passed inside square brackets.
##INDEX 0 1 2
letters = ['a', 'b', 'c']
--------------------------------------------------------------------------
#Print the first element:
print(letters[0])
: 'a'
#Print the third element:
print(letters[2])
: 'c'
#Print the last element:
print(letters[-1]) #Index -1 identifies the last element
: 'c'
#Print the element before the last element:
print(letters[-2])
: 'b'
Accessing items of a list - slicing:
Very often we want to retrieve more than one item from a list: in this case we pass the first index and the last index plus 1 of the item we want to retrieve inside square brackets, with the two integers separated by a colon. Python won't slice the item of the list that has the index equal to the second integer passed. That's why the value index after the colon of second needs to be increased of 1.
#INDEX 0 1 2 3 4 5 6
letters = ['a', 'b', 'c', 'd', 'e', 'f', 'g']
##To access the first 3 elements of the list:
#Option 1:
print(letters[0:3]) #To get item with indexes 0 to 2, we pass 0:3
: ['a', 'b', 'c']
#Option 2:
print(letters[:3])
: ['a', 'b', 'c']
##To access items c, d, e:
print(letters[2:5]) #To get item with indexes 2 to 4, we pass 2:5
: ['c', 'd', 'e']
##To access each element from 'c' to the end of the list:
print(letters[2:])
: ['c', 'd', 'e', 'f', 'g']
##To access the first last 3 elements of the list:
#Option 1:
print(letters[4:7]) #This approach requires knowing the number of items
: ['e', 'f', 'g']
#Option 2:
print(letters[-3:]) #Easier approach
: ['e', 'f', 'g']
##To get every other element of the list:
letters = ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i']
skip_one = letters[0:10:2] #Start:end:step
print(skip_one)
: ['a', 'c', 'e', 'g', 'i']
##To get every 2 elements of the list:
skip_two = letters[0:10:3] #Start:end:step
print(skip_two)
: ['a', 'd', 'g']
Common methods/functions of a list
letters = ['a', 'b', 'c', 'd', 'e', 'f', 'g']
--------------------------------------------------------------------------
##Length of a list = number of items inside a list --> len()
print(len(letters))
: 7
--------------------------------------------------------------------------
##Add an element at the end of a list --> append()
letters.append('h')
print(letters)
: ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h']
--------------------------------------------------------------------------
##Remove an element from a list --> pop()
#If nothing is passed inside the parentheses the last element is removed
letters.pop()
print(letters)
: ['a', 'b', 'c', 'd', 'e', 'f', 'g']
letters.pop(2) #The third element (index = 2) is removed
print(letters)
: ['a', 'b', 'd', 'e', 'f', 'g']
--------------------------------------------------------------------------
##Add an element at a given position --> insert()
letters.insert(2, 'fig') #Insert 'fig' in position with index 2
print(letters)
: ['a', 'b', 'fig', 'd', 'e', 'f', 'g']
--------------------------------------------------------------------------
##Remove a specific element from the list --> remove()
letters.remove('f')
print(letters)
: ['a', 'b', 'fig', 'd', 'e', 'g']
--------------------------------------------------------------------------
##Reverse the elements of the list --> reverse()
letters.reverse()
print(letters)
: ['g', 'e', 'd', 'fig', 'b', 'a']
--------------------------------------------------------------------------
##Return the max and min values of a list --> max(), min()
nums = [2, 7, 1, 23, 0]
print(max(nums)) #To get the max value
: 23
print(min(nums)) #To get the min value
: 0
--------------------------------------------------------------------------
##Sum the elements of a list --> sum()
print(sum(nums))
: 33
--------------------------------------------------------------------------
##Sort the list in ascending order --> sort()
nums.sort()
print(nums)
: [0, 1, 2, 7, 23]
--------------------------------------------------------------------------
##To get the first occurrence of an element --> index()
nums = [1, 7, 0, 7, 23, 40]
print(nums.index(7))
: 1
print(nums.index(23))
: 4
--------------------------------------------------------------------------
##To get the number of occurrences of an element --> count()
nums = [1, 7, 0, 7, 23, 40]
print(nums.count(7))
: 2
--------------------------------------------------------------------------
##To concatenate a list n times --> *
list1 = [1, 7, 0, 'r', 5]
list2 = list1 * 2
print(list2)
: [1, 7, 0, 'r', 5, 1, 7, 0, 'r', 5]
--------------------------------------------------------------------------
##To concatenate two lists together --> extend() #Option 1
list1 = [1, 7, 0, 'r', 5]
list2 = [10, 70, 50]
list1.extend(list2) #List1 is extended with the elements of list2
print(list1)
: [1, 7, 0, 'r', 5, 10, 70, 50]
##To concatenate two lists together --> + #Option 2
list1 = [1, 7, 0, 'r', 5]
list2 = [10, 70, 50]
list3 = list1 + list2 #The concatenation's result is saved in a new list
print(list3)
: [1, 7, 0, 'r', 5, 10, 70, 50]
--------------------------------------------------------------------------
##To erase all the elements from the list --> clear()
list1 = [1, 7, 0, 'r', 5]
print(list1)
: [1, 7, 0, 'r', 5]
list1.clear()
print(list1)
: []
Nested Lists
A nested is a list inside another list. Let's experiment with nested lists.
##Initialize an nested list:
nested_list1 = [[0, 1, 2], [3, 4, 5], [7, 8, 9, 10]]
print(nested_list1)
:[[0, 1, 2], [3, 4, 5], [7, 8, 9, 10]]
##Length of the nested list:
print(len(nested_list1))
: 3 #Nested_list1 contains 3 elements which are 3 lists
##Access the SECOND inner list (index 1):
print(nested_list1[1])
: [3, 4, 5]
##Access the FIRST element of the THIRD list:
print(nested_list1[2][0])
: 7
##Length of the THIRD nested list:
print(len(nested_list1[2]))
: 4
Use case 1
Create a list containing the integers from 0 to 10.
integers = [] #Initialize an empty list where to collect the results
for num in range(11): #The range object won't include 11 !!
integers.append(num)
print(integers)
: [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Use case 2
Given a nested list, flatten it.
nested_letters = [['a', 'b'], ['c', 'd', 'e', 'f', 'g']]
print(nested_letters)
: [['a', 'b'], ['c', 'd', 'e', 'f', 'g']]
print(len(nested_letters)) #Number of elements in the nested list
: 2
flatten_list = [] #Initialize an empty list
for inner_list in nested_letters:
for num in inner_list:
flatten_list.append(num)
print(flatten_list) #Check the result
: ['a', 'b', 'c', 'd', 'e', 'f', 'g']
print(len(flatten_list)) #Number of elements in the flattened list
: 7
Concluding remarks
Lists are easy to use and strategically essential to create complex Python scripts.
Comments